Ryan Collins has some great videos on how to use Perfect to serve web pages and how to use Mustache. These were great for helping me get things going, but I prefer a written format to video, so I am going to repeat a bit of what Ryan has done. Chris Manahan has a good writes up on getting a Perfect project running on how to use MySQL with Perfect so I won't repeat that.
So here we go:
My previous blog post shows how I created classes to serve my MySQL tables as JSON. Here is my
PerfectServerModuleInit Where I add one route to return JSON from my auto_make table.
public func PerfectServerModuleInit() {
I'm creating a web service for my app, but I also want to have a web interface that allows me to administer content for the app. So I need to serve some static pages like my stylesheet to give the look and feel of my service and then of course some dynamic pages.
To get static pages I add a route and connect it to the StaticFileHandler that is part of the PerfectLib.
Now I need to have a file in the webroot. So in my Xcode project I create a group called webroot and add my .css file and background image:

This is great, but to get the server to find these files we need to have them copied into the webroot in the Products Directory. So I select my CarHealthServer project and the Build Phase. Then click on the "+" and add a "New Copy Files Phase"
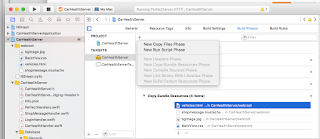
Then I will click on the "+" in the copy files phase and add the files that are in my webroot group. Here I have added a couple of extra files, a vehicles.html and a shop messages.mustache. I'll talk about the .mustache file later.

So now when we run our server and go to http://localhost:8181/BackView.css in my browser I get the contents of my .css file.
I want a page that will let me create an html document that will get saved in a MySQL table. So I create a file call shopmessage.mustache:
The important things here are "{{% handler:ShopMessageHandler}}" which specifies the class that will handle the dynamic content for the mustache file, and "{{doc}}" which is supplied by the handler. Here is my ShopMessageHandler.swift class:
Now I need to modify the PerfectServerModuleInit to register the ShopMessageHandler.
In the valuesForRespose I create a dictionary of the values to be used in the .mustache file. In this case there is just one value and it is hard coded to a static string. But this now gives me the framework to develop my service.
Now when I go to http://localhost:8181/shopmessage.mustache I get this:

So here we go:
My previous blog post shows how I created classes to serve my MySQL tables as JSON. Here is my
PerfectServerModuleInit Where I add one route to return JSON from my auto_make table.
public func PerfectServerModuleInit() {
Routing.Handler.registerGlobally()
// Create Routes
Routing.Routes["/auto_make"] = { _ in return AutoMakeRecord() }
}
To get static pages I add a route and connect it to the StaticFileHandler that is part of the PerfectLib.
public func PerfectServerModuleInit() {
Routing.Handler.registerGlobally()
// Create Routes
Routing.Routes["/*"] = { _ in return StaticFileHandler() }
Routing.Routes["/auto_make"] = { _ in return AutoMakeRecord() }
}

This is great, but to get the server to find these files we need to have them copied into the webroot in the Products Directory. So I select my CarHealthServer project and the Build Phase. Then click on the "+" and add a "New Copy Files Phase"
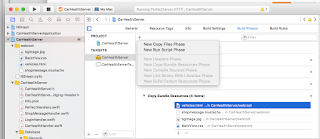
Then I will click on the "+" in the copy files phase and add the files that are in my webroot group. Here I have added a couple of extra files, a vehicles.html and a shop messages.mustache. I'll talk about the .mustache file later.

So now when we run our server and go to http://localhost:8181/BackView.css in my browser I get the contents of my .css file.
I want a page that will let me create an html document that will get saved in a MySQL table. So I create a file call shopmessage.mustache:
The important things here are "{{% handler:ShopMessageHandler}}" which specifies the class that will handle the dynamic content for the mustache file, and "{{doc}}" which is supplied by the handler. Here is my ShopMessageHandler.swift class:
import PerfectLib
class ShopMessageHandler: PageHandler {
func valuesForResponse(context: MustacheEvaluationContext, collector: MustacheEvaluationOutputCollector) throws -> MustacheEvaluationContext.MapType {
var values = MustacheEvaluationContext.MapType()
values["doc"] = "my shop message"
return values
}
}
Now I need to modify the PerfectServerModuleInit to register the ShopMessageHandler.
public func PerfectServerModuleInit() {
PageHandlerRegistry.addPageHandler("ShopMessageHandler") { (r: WebResponse) -> PageHandler in return ShopMessageHandler() }
Routing.Handler.registerGlobally()
// Create Routes
Routing.Routes["/*"] = { _ in return StaticFileHandler() }
Routing.Routes["auto_make"] = { _ in return AutoMakeRecord() }
}
Now when I go to http://localhost:8181/shopmessage.mustache I get this:

No comments:
Post a Comment